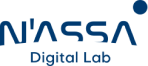


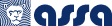
The design of an API is a very important part, with this in mind we design our APIs for easy understanding and use by third parties based on the following principles
Understandable urls based on the purpose of the endpoint.
Filters to improve the API experience and enrichment.
Correct use of HTTP response codes.
Simple identification of errors or incorrect requests.
Unique authentication and authorization to access our different endpoints.
Cache and rate limit management for each of the services we offer.
From now on we will explain in more detail what each element of our API consists of, how to take advantage of them and use them correctly.
A query is a read operation on models that returns a set of data or results. As we introduced in Working with data, repositories add behavior to models. Once you have them set up, you can query instances using a C# API and a REST API with filters as outlined in the following table. Filters specify criteria for the returned data set. The capabilities and options of the two APIs are the same. The only difference is the syntax used in HTTP requests versus Net Core function calls. In both cases, ASSA models return JSON.
Our API supports a specific filter syntax: it’s a lot like SQL, but designed specifically to serialize safely without injection and to be native to Net Core.
API ASSA supports the following kinds of filters:
Fields filter
Include filter
Limit filter
Order filter
Skip filter
Where filter
More additional examples of each kind of filter can be found in the individual articles on filters (for example Where filter). Those examples show different syntaxes in REST.
The following is an example of using the find
method with both a where and a limit filter:
Equivalent using REST: /accounts?filter[where][name]=John&filter[limit]=3
There is no theoretical limit on the number of filters you can apply.
Where:
filterType is the filter: where, include, order, limit, skip, or fields.
spec is the specification of the filter: for example for a where filter, this is a logical condition that the results must match. For an include filter it specifies the related fields to include.
Specify filters in the HTTP query string:
/something?filter=[filterType1]=val1&filter[filterType2]=val2...
or
/something/id?filter=[filterType1]=val1&filter[filterType2]=val2...
The number of filters that you can apply to a single request is limited only by the maximum URL length, which generally depends on the client used.
Important:
There is no equal sign after ?filter
in the query string; for example https://api.assanet.com/something?filter[where][id]=1
Allow selection of a few fields of a resource if not all are required. Giving the API consumer the possibility of which fields they want to be returned reduces the volume of traffic in the communication and increases the speed of using the API.
GET /cars?filter[fields]=manufacturer,model,color
In the example, the list of cars is requested, but asking only the manufacturer, model and color fields.
Allow ascending or descending sorting on multiple fields.
GET /cars?filter[sort]=-manufacturer,+model
The example returns an ordered list of cars with manufacturers in descending order and models in ascending order.
Use of "skip" to set the starting position of a collection and "limit" to set the number of elements of the collection to return from the skip. It is a flexible system for those who consume the API and quite widespread among database systems.
GET /cars?filter[skip]=10&filter[limit]=5
In the example, a list of cars is returned corresponding to the cars comprised from position 10 to 15 of the collection.
It allows including the detail in fields related to the current query and adding the information from another data model within the current request.
GET /cars?filter[include]=driver,owner
The example returns a list of cars including the driver and owner data.
Use a single query parameter for all fields or a formalized query language for filtering.
GET /cars?filter[where][color]=red&filter[where][seat][gt]=2
The example returns a list of red cars with more than two seats.
© 2020 ASSA Compañía de Seguros, S.A. | Todos los derechos reservados. Terms and Conditions